Tried this but hade problem with SaveChanges() probably because we didnt have any XML in XSAttribute column
The problem was that for metaField type “CheckboxBoolean” there was no attribute with the “Label” key. I decompiled the Commerce.UI.Admin DLL and noticed in GetData it was looking for the “Label” key. This was null in my case. I wrote the following code to traverse the metaFields and add the key to that field type.
//MY CODE
using (MetaClassManagerEditScope scope = dataContext.MetaModel.BeginEdit())
{
foreach (MetaClass metaClassLoop in dataContext.MetaModel.MetaClasses)
{
foreach (MetaField field in metaClassLoop.Fields)
{
if (!field.Attributes.ContainsKey("MaxLength"))
{
field.Attributes.Add(McDataTypeAttribute.StringMaxLength, 40000);
}
if (field.TypeName == "CheckboxBoolean" && !field.Attributes.ContainsKey("Label"))
{
field.Attributes.Add(McDataTypeAttribute.BooleanLabel, field.Name);
}
}
}
scope.SaveChanges();
}
As we see in the table, we have NULL in XSAttribute, so we needed to add some XML to that field, we accomplished that with this MigrationStep:
using System;
using EPiServer.DataAbstraction.Migration;
using EPiServer.ServiceLocation;
using Microsoft.Data.SqlClient;
using Microsoft.Extensions.Configuration;
namespace Gosso.Bug.Workarounds
{
/// <summary>
/// missing "Label" in database, this can be removed after run once.
/// </summary>
public class AddMissingAttributesMigrationStep : MigrationStep
{
public override void AddChanges()
{
string connectionString = ServiceLocator.Current.GetInstance<IConfiguration>().GetConnectionString("EPiServerDB");
bool runOnce = false;
string query = @"
SELECT [XSAttributes]
FROM [X.Commerce].[dbo].[mcmd_MetaField]
WHERE typename = 'CheckboxBoolean' AND metaclassid = 3 AND [XSAttributes] IS NULL;
";
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
using (SqlCommand command = new SqlCommand(query, connection))
{
using (SqlDataReader reader = command.ExecuteReader())
{
if (reader.HasRows)
{
runOnce = true;
}
else
{
Console.WriteLine("No rows found with NULL XSAttributes values.");
}
}
}
}
if (runOnce)
{
query = @"
UPDATE [X.Commerce].[dbo].[mcmd_MetaField]
SET XSAttributes = '<?xml version=""1.0"" encoding=""utf-8""?>
<AttributeCollection>
<Attr>
<Name>Label</Name>
<Type>System.String, System.Private.CoreLib</Type>
<Value><string>True</string></Value>
</Attr>
<Attr>
<Name>MaxLength</Name>
<Type>System.Int32, System.Private.CoreLib</Type>
<Value>
<int>40000</int>
</Value>
</Attr>
</AttributeCollection>'
WHERE typename = 'CheckboxBoolean' AND metaclassid = 3";
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
using (SqlCommand command = new SqlCommand(query, connection))
{
int rowsAffected = command.ExecuteNonQuery();
}
}
}
}
}
}
No that didnt work, as we dont know the names in DXP.
we emailed support to run these two scripts: (to add the missing xmls in XSAttribute)
SQL Script 1:
UPDATE [CHANGETHIS.Commerce].dbo.mcmd_MetaField SET XSAttributes = '<?xml version="1.0" encoding="utf-8"?><AttributeCollection><Attr><Name>Label</Name><Type>System.String, System.Private.CoreLib</Type><Value><string>True</string></Value></Attr><Attr><Name>MaxLength</Name><Type>System.Int32, System.Private.CoreLib</Type><Value><int>40000</int></Value></Attr></AttributeCollection>'
SQL Script 2:
UPDATE [CHANGETHIS.Commerce].dbo.mcmd_MetaField SET XSAttributes = '<?xml version="1.0" encoding="utf-8"?><AttributeCollection><Attr><Name>MaxLength</Name><Type>System.Int32, System.Private.CoreLib</Type><Value><int>40000</int></Value></Attr></AttributeCollection>'
WHERE typename = 'Text' and XSAttributes is Null
Hi
We updated from 14.5 to latest 14.15.2.
we get
System.NullReferenceException: Object reference not set to an instance of an object.
at lambda_method404(Closure , Object , Object[] )
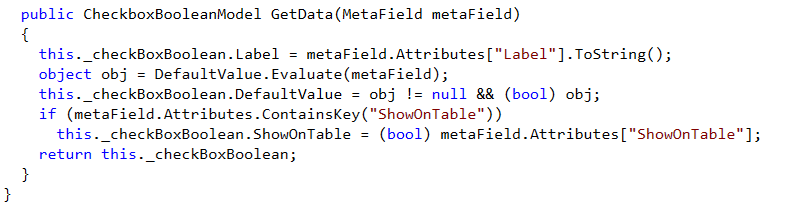
What is Label here: Probably it does not exist in our db?? why not use ContainsKey here?
Database looks like this:
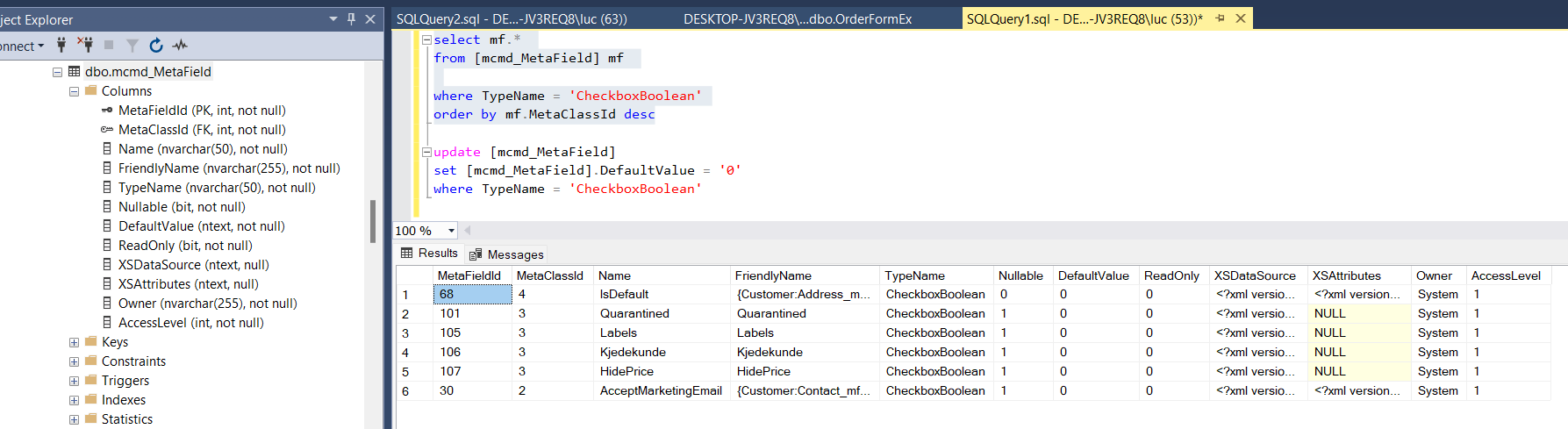
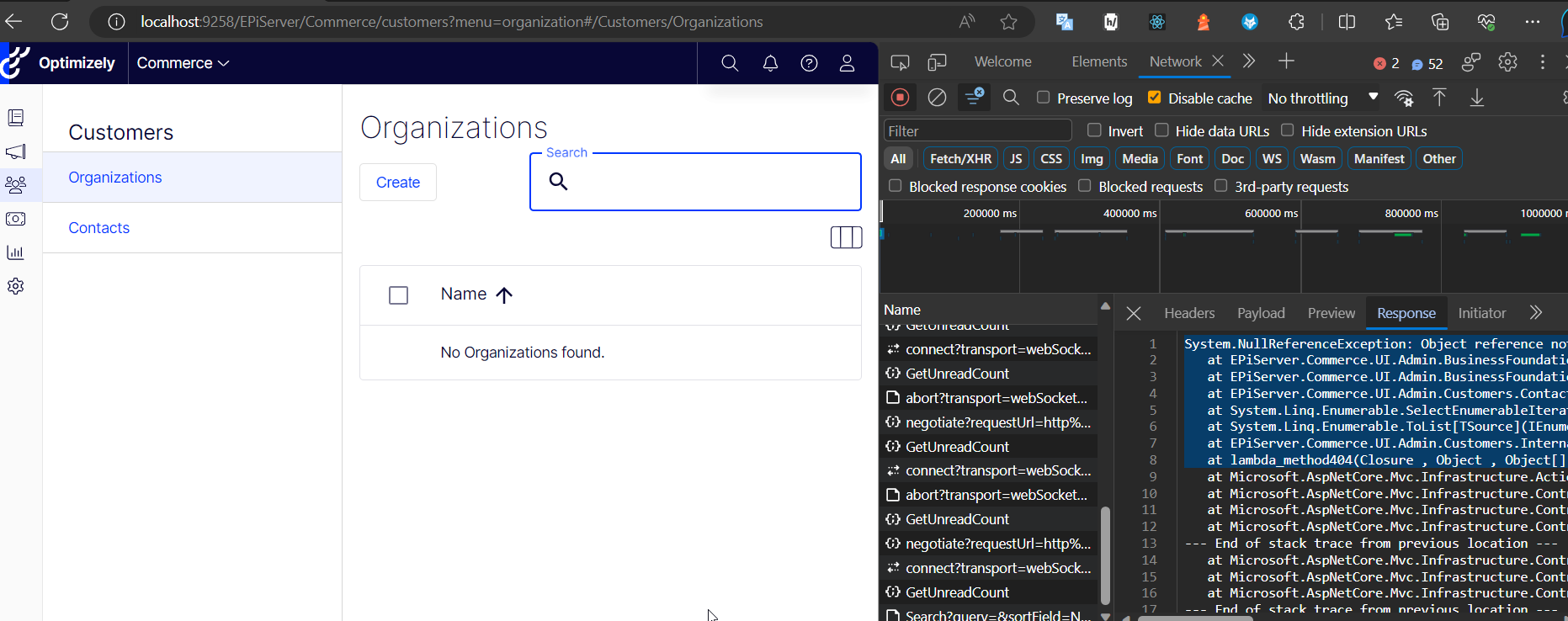
no contact, no orgs, error above